14 Feb 2019
C pointer is powerful that can point to anywhere with good definition. Here are collections of interesting way to use pointer.
definition |
meaning |
link |
type *i; |
simple pointer |
here |
type *i[n]; |
array of n pointer |
here |
type (*p)[n]; |
a pointer to an array of n |
here |
type (*p)(); |
a pointer to function that return type |
here |
type (*p[n])(); |
a pointer to an array of n functions that return type |
here |
type *i
int j;
int *i;
i = &j;
*i = 10;
// j == 10
type *i[n]
int j;
int *i[10];
i[0] = &j;
*i[0] = 10;
// j == 10
type (*p)[n]
int i[10];
int (*p)[10];
p = &i;
*p[0] = 10;
*((*p)+1) = 11;
// i = {10,11,?,...,?}
type (*p)()
int test() {
printf("%s\n", "test");
return 1;
}
int main() {
int (*x)();
x = &test;
int z = x();
printf("%d\n", z);
}
//result:
//test
//1
type (*x[n])()
int test() {
printf("%s\n", "test");
return 1;
}
int main() {
int (*x[10])();
x[0] = &test;
int z = x[0]();
printf("%d\n", z);
}
//result:
//test
//1
06 Feb 2019
This page is intended to show how I do during a social engineering phishing in EECS3482 at York University. This is not going to record any of the data you put in the form.
The process is simple, just download the whole page and modify the form. and it is done. It requires some HTML skill to understand how it works.
This is kind of attack that trick user put their password into a fake login form. And you will see your password in the URL bar in this case. That way, the attacker will record the password and find a chance to steal your account.
There are many ways to prevent. Simply look closely at the URL. Does it look different or is it SSL protected with a green lock and the name of the company.
05 Feb 2019
I am trying to build a step counter with MPU6050, and it is not so hard to do it. I learn a lot of stuff relative to how hardware like arduino and other parts communicating.
Type of communication
I found three common type of communication protocals are widely used:
- I2C (Inter-Integrated Circuit Protocol)
- UART (Universal Asynchronous Receiver/Transmitter)
- SPI (Serial Peripheral Interface)
I2C (Inter-Integrated Circuit)
This protocal is simple to use. It connects all part via I2C Bus which consist two lines are Serial Data Line (SDA) and Serial Clock Line (SCL).
A microcontroller act as master and other part act as slave takes controll from the master. The clock is controll by the master. Sameple schematic below, from wikipedia.
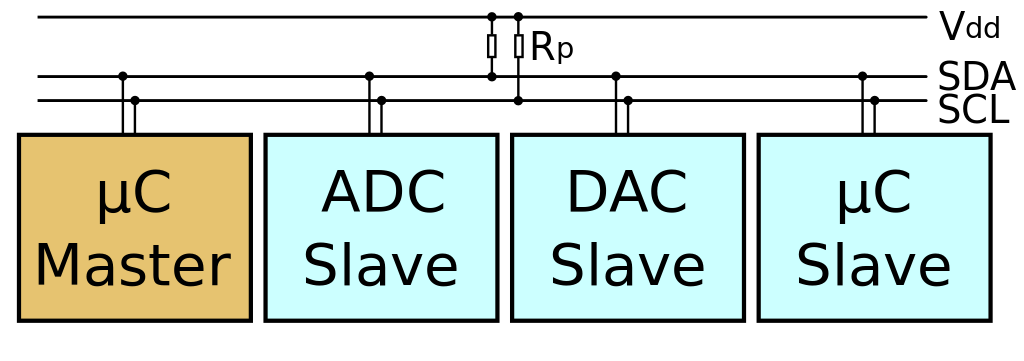
Each device have a unique address so thry can communicate. Basic communication is using transfers of 8 bytes. Each I2C slave have 7 bytes address. The bit 0 is signal to read or write to the device.
Communication is initialized by master device. In normal state, both lines SCL and SDA are high. Then Start condition is drop the SDA line. After that, ther master will start sending the address. Then follow by one bit of operation. If bit was 0, it means writing to slave. If bit was 1, then it will read from the slave. Once all bits are finish, it follows the Stop condition. Then the other device on the bus can use the bus. The image show how the device communicate, image from here.

UART (Universal Asynchronous Receiver/Transmitter)
This is not really a kind of communication protocol like I2C. It is a standalone IC. It only use two wires (two Tx pin to Rx pin) to transmit data between devices. This transmit data asynchronously, because there is no clock needed. Instead, it adds start and stop bit to the packet (Image below from here). So, it know when to start reading and end reading. They read byte at a specify frequency aka baud rate. Baud rate is the speed of data express in bit pre second (bps).
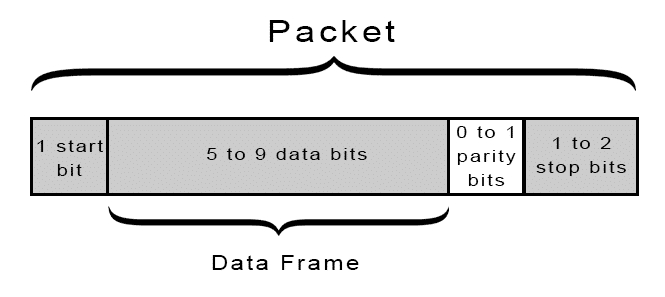
It works simple, however, you cannot connect multiple slave or mutiple master systems. Also, we need to adjuest the BPS to match the other part.
It’s no compatible with the I2C.
SPI (Serial Peripheral Interface)
I haven research about this. I have little knowledge of it. Thats how arduino and computer communicate. I will do some more research about it.
20 Jan 2019
I was trying to make a page with a full image background. It was simple. I fit everything into one div
with class container. And give the background to the body tag.
body {
background-image: url(/image/bg.jpg);
background-repeat: no-repeat;
background-size: cover;
}
It works well. However, I tried to make it responsive to different devices. It leaves an ugly white space at the bottom. I can tell that it is because the body did not have a value of height. I have to force it to have the height of the screen. Then, the code becomes:
body {
height: 100%;
background-image: url(/image/bg.jpg);
background-repeat: no-repeat;
background-size: cover;
}
It actually overdoes it. I now have a scroller at the right. This is so confusing for the unexpected result. After researching a little bit. The margin
value actually pull the body down, so I got a margin for the body at the top.
There is a simple fix. I just add one more div
that warps container, and give it overflow: auto;
.
.page {
overflow: auto;
}
07 Jan 2019
In GNU C Library, there are ways to Customizing printf.
It allows you to create conversion specifiers. It allow you to print important data structures of our programe.